As you are aware, Node.js is utilized to create server-side applications using JavaScript and supports HTTP requests, making it a robust platform for developing web applications that depend significantly on server-side communication and data transfer. For most developers, making HTTP requests in Node.js is a crucial task.
Although it appears straightforward, there are several essential aspects to understand, especially the various methods of making HTTP requests. Today, let's discuss how different packages such as Axios, Got, Node-Fetch, Request-Promise, Simple-Get, SuperAgent, and the HTTPS module can be used for HTTP requests.
To better understand our program, we will test it by making GET requests to a mock API using all available HTTP client options. This approach will ensure that the program operates correctly and can handle different request types and configurations. Use this mock API for reference:
Prerequisites
Before we dive in, it’s good to get ready with the following prerequisites you’ll need to try the shared code.
- Install a Node.js runnable environment on your machine. Make sure you have latest versions of Node.js and npm installed.
- The npm commands are used to install the required package which in return makes our development process easier with specific requests over a server. You can use npm init command to initialize node package and npm install --save <module-name> to initialize it, for your project.
- If you want to run Javascript files, use node <file_name> on your compound line and get the desired output in your code editor console.
HTTP(S) - Node.js Standard library
Getting started with HTTP(S) module, is absolutely hassle free. It is a pre-installed module that comes with Node.js bundle. It is used by many Node.js projects by default, including popular frameworks like Express and Koa. often use it as a reference point for comparing and evaluating other HTTP request libraries for Node.js.
To understand more about HTTP(S)-Node.js Standard Library, let’s look at this mock Zipy API code snippet to send a GET request:
Let's break down how the code functions:
- Imports the HTTP(S) module: The built-in HTTP(S) module in Node.js is imported.
- Creates an options object: This object contains essential details to make the HTTP request, such as the server's hostname, the API endpoint's path, and the HTTP method.
- Initiates an HTTP request: Using the 'https.request()' method, an HTTP request is created, incorporating the previously defined options object. Additionally, a callback function is set up to handle the server's response.
- Asynchronous nature: The request() method in these modules operates asynchronously, ensuring the main execution thread remains unblocked during the request process.
- Error handling and request completion: Potential errors during the HTTPS request can be managed, and the request is concluded using the 'req.end()' method, which dispatches the request to the server.
Axios
Axios is a versatile HTTP client that works seamlessly in both browser and Node.js environments. It simplifies the process of sending asynchronous HTTP requests to REST API endpoints, making it easy to perform CRUD operations. Axios automatically converts request and response data to JSON, streamlining data handling. On the client side, Axios supports HTTP/2, enhancing performance.
As of April 2023, the Axios GitHub repository boasts over 85,000 stars and 9,300 forks, highlighting a large and active community of users and contributors. Axios is utilized by notable Node.js projects like the Vue and Nuxt frameworks, the Redux state management library, and the Gatsby static site generator.
To install Axios, use the npm command:
Accomplish your process with Axios:
Here's an explanation of how the code functions:
- Making the Request: We use the
get
method from the Axios library to send an HTTP GET request to the endpoint 'https://api.zipy.ai/services/errors', including a query parameter 'api_key' with the value 'DEMO_KEY'. - Handling the Response: Once the server responds, the code logs the HTTP status code to the console with
response.status
, and it also logs the response data usingresponse.data
. - Error Handling: If an error occurs during the HTTP request, the
catch
block captures it and logs the error to the console usingconsole.error()
.
In summary, this code makes a GET request to a mock Zipy API to retrieve error information, utilizing a demo API key.
Got
Got library is a popular HTTP request library for Node.js which helps developers send HTTP requests and handle responses. Its simplified API promises usability and error handling, makes it easy to use. It provides a slimmed-down version of the requests package. It also has a promise-based API, HTTP/2 support, and a pagination API, which are unique to the Got library. The GitHub repository for Got library has gained 17,000 stars and 1,200 forks, as of April 2023.
Install Got using npm command:
Here’s the code for using Got:
Here's an explanation of how the code operates:
- Importing the Library: The "got" library is imported and assigned to a variable named
got
. - Sending the GET Request: The "got" library is used to send an HTTP GET request to the '/services/errors' endpoint of the Zipy API. The request options are provided as an object with the following properties:
searchParams
: Contains the query string parameters for the HTTP request.responseType
: Specifies the expected response type, which in this case, is JSON.
- Handling a Successful Response: The
.then()
method is called on the returned promise from the "got" request. This method takes a callback function that executes if the request is successful. The callback function receives the HTTP response object as its argument. Inside this function, the response's status code and body are logged to the console usingconsole.log()
. - Handling Errors: The
.catch()
method is also called on the promise object. It takes a callback function that executes if an error occurs during the request. This function receives an error object as its argument, and the error is logged to the console usingconsole.error()
.
In summary, this code sends a GET request to the Zipy API to fetch error information, logs the response if successful, and handles any errors that might occur.
Node-Fetch
Node-Fetch library is a light wrapper HTTP request library which brings the browser window.fetch method to Node.js. It provides a consistent and easy-to-use API for making HTTP requests in Node.js, with a focus on native promises, and async/await syntax along with window.fetch.
Metrics for Node-Fetch suggest it as a widely used and popular HTTP request library, as it has 15,000 stars and 1,000 forks, as of April 2023.
Install Node-Fetch using npm command:
Check out how node-fetch can be used to call mock Zipy API:
Here’s a breakdown of how the above code works:
- Import the 'node-fetch' library and assign it to a variable named 'fetch'. Send an HTTP GET request to the URL using it.
- Handle the HTTP response information by calling .then() method on the promise. There are two “.then()” method, the first one takes a function as a parameter which gets executed when the promise is resolved. The second one takes the 'response' object as a parameter, which contains the HTTP response information.
- The 'response.status' property contains a status code which is printed as console.log() function.
- To parse the response body as JSON, apply '.json()' method on the response object.
- The second .then() method is called on the promise returned by '.json()' to handle the parsed JSON data. The method takes a function as a parameter that will be executed when the promise is resolved. The function takes the parsed JSON data as a parameter.
- The 'console.log()' method is used to log the parsed JSON data to the console.
- .catch() method is called on the promise to handle any errors that may occur during HTTP request or parsing of the response body. Finally, we log the error object to the console using 'console.error()' method.
Request-Promise
Request-Promise library allows HTTP requests in Node.js to be made using promises. It is powered by Bluebird. You will see, how it adds Bluebird powered .then(…) method to the request call objects. With a moderately sized community of users and contributors, the Request-Promise 1,900 stars and 220 forks, as of April 2023.
Install Request-Promise using npm command:
Below snippet will send a GET request to the mock Zipy API:
Here’s a breakdown of how the above code works:
- Just like all other modules, the code starts with an import of the library and assigns it to a variable named rp.
- The options object is defined, which contains the configuration options for the HTTPS request. The uri property specifies the URL that the request should be sent to, and the qs property is an object that contains the query string parameters to be sent with the request. In this case, the api_key parameter is set to 'DEMO_KEY'. Set the json property to 'true', which specifies that the response should be parsed as JSON.
- After that, the rp function sends a HTTPS GET request to the URL specified in the options object. It will return a promise that resolves the HTTP response information.
- Now, we call .then() method on the promise to handle the HTTP response information.
- Here, console.log() method is used to log the response object to the console. It will then parse the JSON response data, since the json property of the options object was set to true.
- Now, you can call the .catch() method on the promise to handle any errors that may occur and log the error object to the console.
Simple-Get
Simple-Get library is a lightweight HTTP request library for Node.js that makes it easy to send HTTP requests and handle responses. It is a library which is more efficient and has less code. Similar to other libraries, Simple-Get allows redirects and composes well with npm packages for features like cookies, proxies, form data, & OAuth.
Compared to the above libraries, Simple-Get library is relatively less popular with 240 stars and 29 forks on its github repository.
Install Simple-Get using this npm command:
Below code will explain how Simple-Get accomplish the request:
Here’s a breakdown of how the above code works:
- The code imports the "simple-get" library and assigns it to a variable named 'simpleGet'.
- Defines a variable named 'url' including the 'api_key' parameter with a value of 'DEMO_KEY'.
- Sends HTTP GET request to the URL
- Here, the callback function takes two parameters, 'err' and 'response'. If an error occurs during the HTTP request, 'err' will contain the error information. If the request is successful, "response" will contain the HTTP response information.
- If err is not null, then there has been an error. If the error is null, then the request was successful, and the console.log() method logs the status code of the answer.
- 'response.pipe()' method is called on the response object to pipe the response to the standard output stream. Then, the response is logged to the console.
SuperAgent
The SuperAgent package is a well-known HTTP request library for Node.js that makes it simple to send HTTP requests and manage results. It is similar to Axios which is primarily used for making AJAX requests in the browser as well as in Node.js. SuperAgent, lets you chain onto requests such as query() to add parameters to the requests. Earlier, developers had to manually add them in the URL.
SuperAgent is used on a large scale and has a active community of users and contributors scaling its Github repository to 13,000 stars with 1,200 forks in the latest report of April 2023.
Install SuperAgent using npm command:
Run through this code snippet to understand how SuperAgent functions:
Here’s a breakdown of how the above code works:
- Import 'Superagent' library and assign it to a variable named request. Now define a variable named url that contains the URL of the Zipy API's '/services/errors' endpoint.
- The request object is used to send an HTTP GET request to the 'url' variable value, which is the “/services/errors” endpoint of the Zipy API. The request query string parameters are set using the .query() method, which takes an object containing the 'api_key' parameter with a value of 'DEMO_KEY'. The 'end()' method is then used to send the request and take a callback function as a parameter.
- The callback function takes two parameters, err and res. If an error occurs during the HTTP request, first parameter will contain the error information. If the request is successful, second parameter will contain the HTTP response information.
- If an error is not null, the code reports it to the console using the 'console.error()' method, and if it is null, it is logged to the console using the console.log() function in the body of the response.
Most popular library to make HTTP request
To find out which Node.js HTTP client library is winning the most hearts, we went to npm trends, and checked the downloads in the last one year for Axios, Got, Node-Fetch. Request-Promise, Simple-Get, and SuperAgent. If you are wondering why did we skipped HTPPS standard Node library, the answer is - it is already a part of the Node.js package and gets downloaded with the Node bundle.
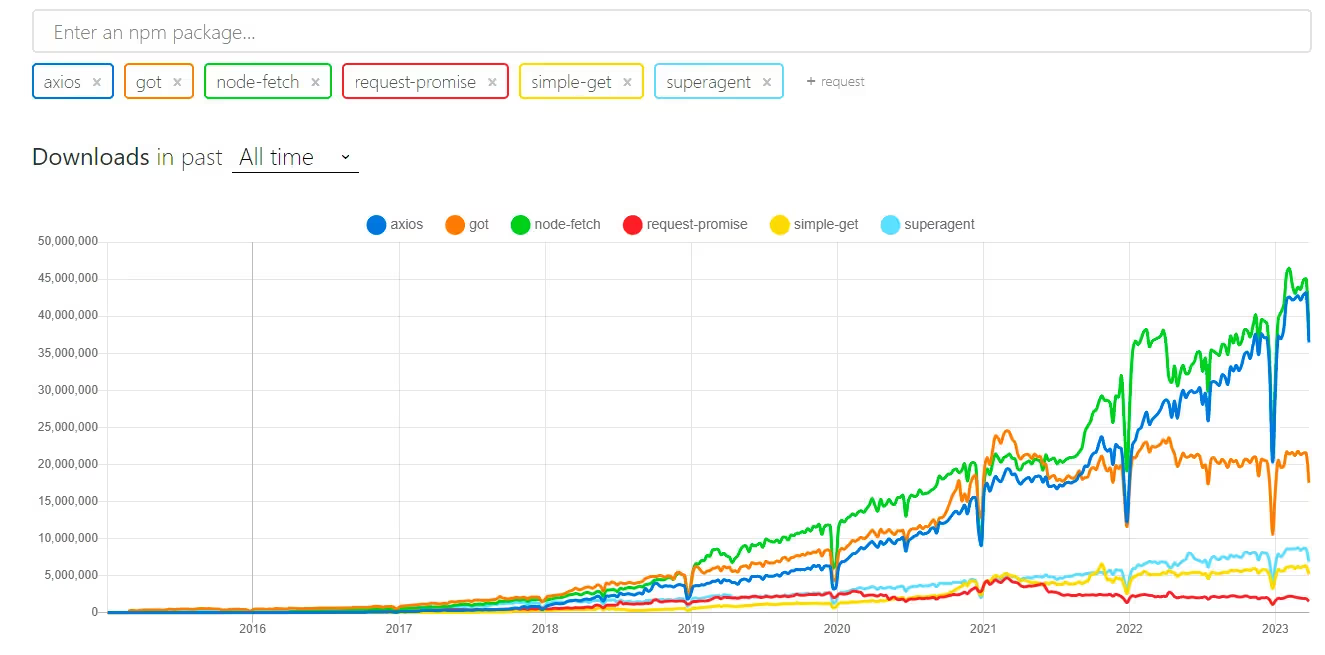
It is crystal clear in terms of downloads, Node-Fetch is the most popular, and Request-Promise is least popular. Node-Fetch has made HTTPS requests more efficient and fast, which rightfully earns the spot. All the HTTP requests libraries give a great deal of freedom in how requests and responses are handled.
Conclusion
Node.js offers several ways to make HTTP requests to web servers and API’s, each with its own advantages and use cases. All the above libraries mainly do the same thing - much like changing the quantity of ingredients in the same recipe.
Ultimately, choosing one method over the other will mostly depend on the specific requirements of the project at hand, like ease of use, performance, security, and compatibility with existing code. We hope this list helped you understand the different methods for making HTTP requests In Node.js and pick the one that’s right for your project.
Happy Coding!